http://sir.kr/so_app/1244 에 있는 내용입니다.
아래와 같이 만들어 보는 것 입니다.
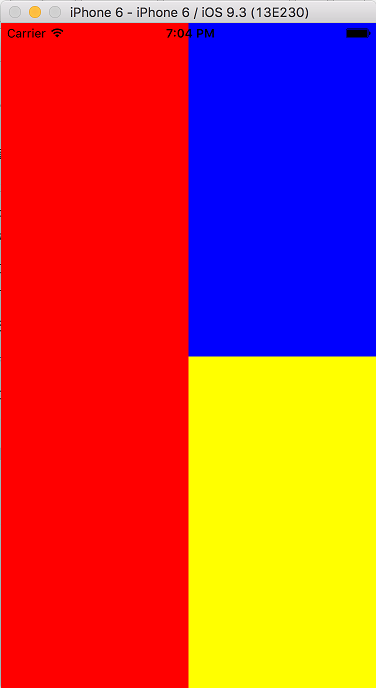
import React, { Component } from 'react';
import { View, StyleSheet, AppRegistry } from 'react-native';
class gitbookTest extends Component {
render() {
return (
<View>
</View>
}
}
}
const styles = StyleSheet.create({
});
AppRegistry.registerComponent('gitbookTest', () => gitbookTest);
위의 코드는 기본 골격입니다. 이 안에 코드를 넣어서 위와 같이 만들어 보세요.
크게 가로, 세로로 1대1이고(left, right), 오른쪽은 위, 아래 1대1로(rightTop, leftTop)
https://cdn.rawgit.com/dabbott/react-native-web-player/gh-v1.6.1/index.html#platform=ios&code=import%20React%2C%20%7B%20Component%20%7D%20from%20%27react%27%3B%20%0Aimport%20%7B%20View%2C%20StyleSheet%2C%20AppRegistry%20%7D%20from%20%27react-native%27%3B%0A%20%0Aclass%20gitbookTest%20extends%20Component%20%7B%0A%20%20render%28%29%20%7B%0A%20%20%20%20return%20%28%0A%20%20%20%20%20%20%3CView%3E%0A%20%20%20%20%20%20%3C/View%3E%0A%20%20%20%20%29%0A%20%20%7D%0A%7D%0Aconst%20styles%20%3D%20StyleSheet.create%28%7B%0A%7D%29%3B%0AAppRegistry.registerComponent%28%27gitbookTest%27%2C%20%28%29%20%3D%3E%20gitbookTest%29%3B%20
먼저 left right를 나눠 보시죠..
class gitbookTest extends Component {
render() {
return (
<View style={styles.container}>
<View style={styles.left}>
</View>
<View style={styles.right}>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
},
left: {
flex: 1,
backgroundColor: 'red'
},
right: {
flex: 1,
flexDirection: 'column',
},
});
위의 코드를 카피해서 넣어 보세요
왼쪽에 빨간색이 나왔나요?
다음은 오른쪽을 2칸으로 나눠야 겠죠.. 방향을 column으로 하고 righTop, rightBottom
class gitbookTest extends Component {
render() {
return (
<View style={styles.container}>
<View style={styles.left}>
</View>
<View style={styles.right}>
<View style={styles.rightTop}>
</View>
<View style={styles.rightBottom}>
</View>
</View>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: 'row',
},
left: {
flex: 1,
backgroundColor: 'red'
},
right: {
flex: 1,
flexDirection: 'column',
},
rightTop: {
flex: 1,
backgroundColor: 'blue'
},
rightBottom: {
flex: 1,
backgroundColor: 'yellow'
}
});
제대로 나왔나요?
https://cdn.rawgit.com/dabbott/react-native-web-player/gh-v1.6.1/index.html#platform=ios&code=import%20React%2C%20%7B%20Component%20%7D%20from%20%27react%27%3B%0Aimport%20%7B%20View%2C%20StyleSheet%2C%20AppRegistry%20%7D%20from%20%27react-native%27%3B%0A%0Aclass%20gitbookTest%20extends%20Component%20%7B%0A%20%20render%28%29%20%7B%0A%20%20%20%20return%20%28%0A%20%20%20%20%20%20%3CView%20style%3D%7Bstyles.container%7D%3E%0A%20%20%20%20%20%20%20%20%3CView%20style%3D%7Bstyles.left%7D%3E%0A%20%20%20%20%20%20%20%20%3C/View%3E%0A%20%20%20%20%20%20%20%20%3CView%20style%3D%7Bstyles.right%7D%3E%0A%20%20%20%20%20%20%20%20%20%20%3CView%20style%3D%7Bstyles.rightTop%7D%3E%0A%20%20%20%20%20%20%20%20%20%20%3C/View%3E%0A%20%20%20%20%20%20%20%20%20%20%3CView%20style%3D%7Bstyles.rightBottom%7D%3E%0A%20%20%20%20%20%20%20%20%20%20%3C/View%3E%0A%20%20%20%20%20%20%20%20%3C/View%3E%0A%20%20%20%20%20%20%3C/View%3E%0A%20%20%20%20%29%3B%0A%20%20%7D%0A%7D%0A%0A%0Aconst%20styles%20%3D%20StyleSheet.create%28%7B%0A%20%20container%3A%20%7B%0A%20%20%20%20flex%3A%201%2C%0A%20%20%20%20flexDirection%3A%20%27row%27%2C%0A%20%20%7D%2C%0A%20%20left%3A%20%7B%0A%20%20%20%20flex%3A%201%2C%0A%20%20%20%20backgroundColor%3A%20%27red%27%0A%20%20%7D%2C%0A%20%20right%3A%20%7B%0A%20%20%20%20flex%3A%201%2C%0A%20%20%20%20flexDirection%3A%20%27column%27%2C%0A%20%20%7D%2C%0A%20%20rightTop%3A%20%7B%0A%20%20%20%20flex%3A%201%2C%0A%20%20%20%20backgroundColor%3A%20%27blue%27%0A%20%20%7D%2C%0A%20%20rightBottom%3A%20%7B%0A%20%20%20%20flex%3A%201%2C%0A%20%20%20%20backgroundColor%3A%20%27yellow%27%0A%20%20%7D%0A%0A%7D%29%3B%0AAppRegistry.registerComponent%28%27gitbookTest%27%2C%20%28%29%20%3D%3E%20gitbookTest%29%3B
댓글 0개